As an iOS developer, understanding the foundations of Core Data coding is crucial for building robust and data-driven applications. Core Data is a powerful framework provided by Apple that allows developers to manage complex object graphs and persist data effortlessly. In this article, we will explore the ins and outs of Core Data coding, covering everything from creating data models to fetching and manipulating data. Join us on this journey to unlock the full potential of Core Data!
Core Data: An Overview
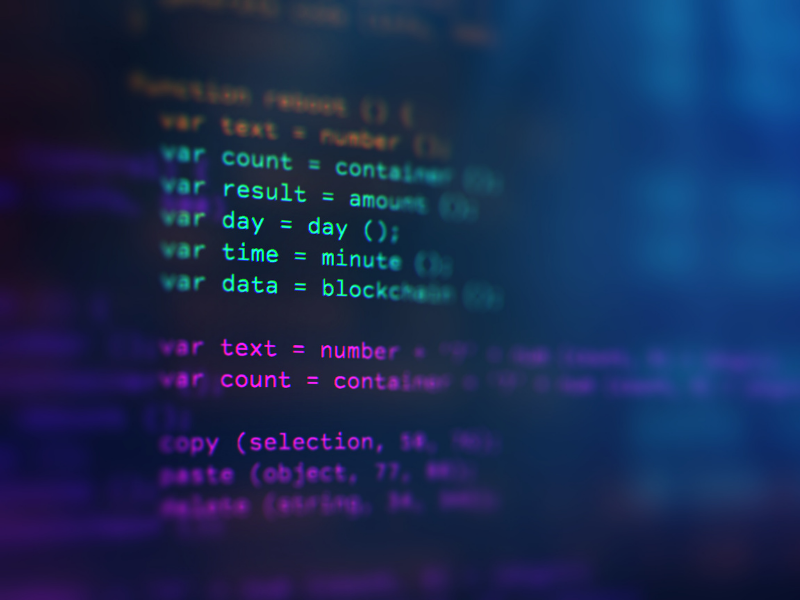
Before diving into the coding aspects of Core Data, let’s take a moment to understand what it is and why it is widely used in iOS development.
What is Core Data?
Core Data is a framework provided by Apple that allows developers to manage the model layer objects in an application. It provides a set of tools and APIs to deal with object storage, retrieval, and manipulation. Core Data is not just a simple database, but a full-fledged object graph management framework that includes features like validation, faulting, undo/redo support, and more.
Why use Core Data?
Using Core Data in your iOS applications offers numerous benefits, including:
- Efficiency: Core Data optimizes data access and storage, resulting in faster and more efficient operations.
- Memory Management: Core Data handles memory management by faulting objects, meaning it only fetches data when needed, saving precious system resources.
- Undo/Redo Support: Core Data provides built-in support for undo and redo operations, making it easier to handle user interactions and data changes.
- Relationship Management: Core Data simplifies managing relationships between objects, allowing you to establish and maintain complex data models effortlessly.
- Thread Safety: Core Data ensures high-level thread safety, providing mechanisms to manage concurrent data access and updates.
Now that we have a good understanding of Core Data and its advantages, let’s dive into the coding aspects.
Creating a Core Data Model
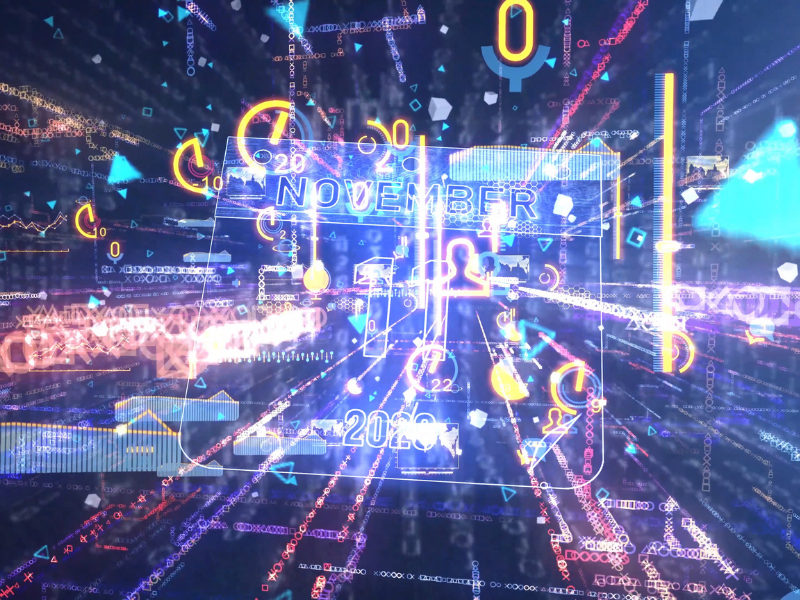
To start using Core Data in your app, you need to define a data model that represents the objects you want to manage. Let’s explore how to create a Core Data model in Xcode and define entities, attributes, and relationships.
Creating a Data Model
- Open Xcode and create a new project or open an existing one.
- Go to the project navigator, right-click on the project folder, and select “New File.”
- Choose “Data Model” under the “Core Data” section and give it a meaningful name.
- Xcode will generate a
.xcdatamodeld
file for you to work with.
Defining Entities
Within the data model, you can define entities, which represent the objects you want to store in your app. Each entity can have attributes and relationships associated with it.
To add an entity:
- Select the
.xcdatamodeld
file in Xcode. - Click on the “+” button at the bottom-left corner of the editor window.
- Enter a name for the entity and press Enter.
Adding Attributes and Relationships
Entities can have attributes that define their properties, such as name, age, or email. Additionally, entities can have relationships with other entities, allowing you to establish connections between objects.
To add attributes and relationships:
- Select the entity you want to add attributes or relationships to.
- Click on the “+” button under the entity.
- Choose either “Attribute” or “Relationship” from the dropdown menu.
- Fill in the details for the attribute or relationship and press Enter.
Fetching and Manipulating Data in Core Data
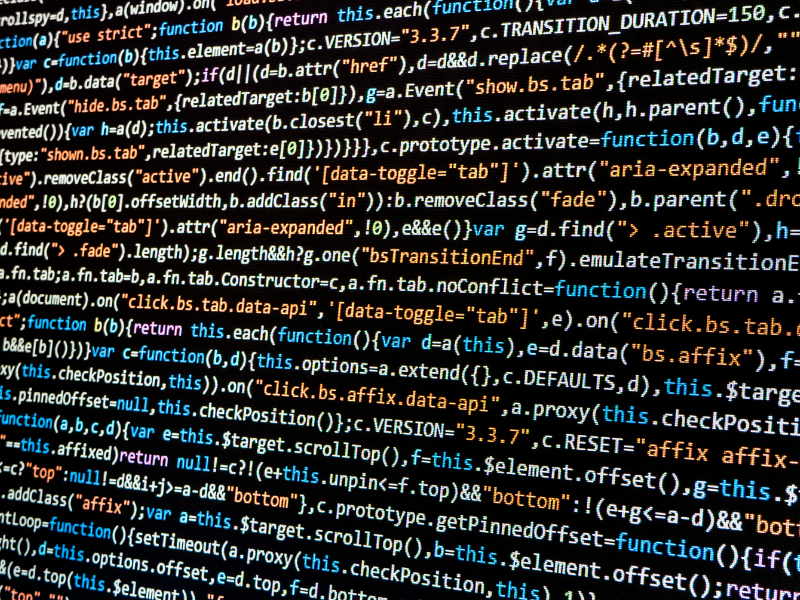
Now that we have our data model set up, let’s explore how to fetch and manipulate data using Core Data coding.
Fetching Data
Fetching data from Core Data involves constructing fetch requests and executing them to retrieve the desired objects. With Core Data, you can filter, sort, and limit the fetched results.
To fetch data from Core Data, follow these steps:
- Create a fetch request using
NSFetchRequest
. - Set the entity to fetch and any predicates, sort descriptors, or fetch limits if needed.
- Execute the fetch request using
NSManagedObjectContext
.
Manipulating Data
Core Data provides methods to insert, update, and delete objects within the managed object context. To manipulate data, follow these steps:
- Retrieve the managed object context associated with your data model.
- Create a new managed object using
NSEntityDescription
. - Set the attribute values for the newly created object.
- Save the changes to persist them using
NSManagedObjectContext
.
FAQs
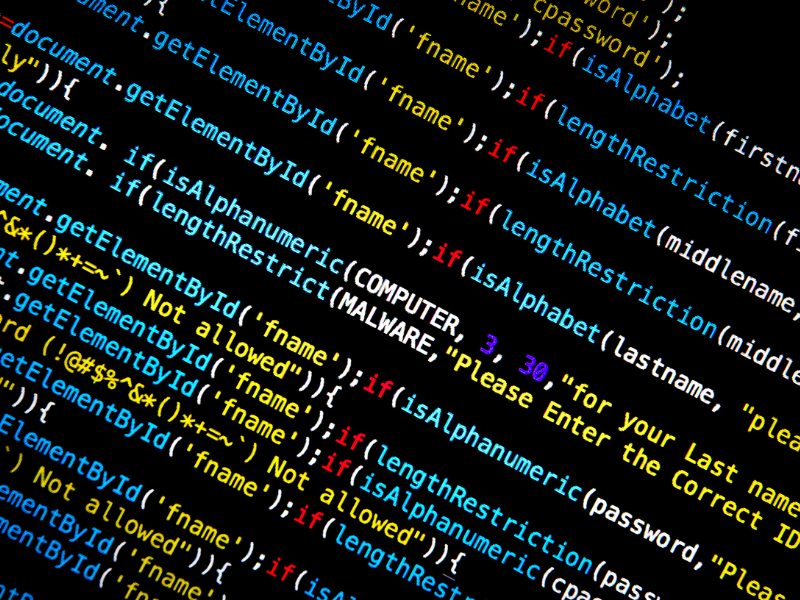
Q: Can I use Core Data in non-iOS projects?
A: Yes, Core Data is not limited to iOS projects. It is available on macOS, watchOS, and tvOS as well, allowing you to leverage its power across different Apple platforms.
Q: Are there any alternatives to Core Data for managing data in iOS apps?
A: Yes, there are alternatives to Core Data, such as Realm and SQLite. Each has its advantages and trade-offs, so it’s essential to evaluate your project’s requirements before choosing the best option.
Q: Does Core Data support the migration of data models?
A: Yes, Core Data provides migration options to handle changes in your data model over time. It supports lightweight and heavyweight migration, making it easier to evolve your app’s data structure without losing existing data.
Q: Can I use Core Data with SwiftUI?
A: Absolutely! Core Data seamlessly integrates with SwiftUI, allowing you to build modern and data-driven apps with a scalable data storage solution.
Conclusion
Core Data coding is an essential skill for iOS developers who want to build powerful and efficient applications. Understanding the fundamentals of Core Data, such as creating data models and manipulating data, is crucial for leveraging the full potential of this framework. By following the guidelines outlined in this comprehensive guide, you’ll be well-equipped to tackle any Core Data coding challenge and deliver exceptional user experiences.