Introduction
SwiftUI is a cutting-edge framework introduced by Apple that revolutionizes the way developers build user interfaces for iOS, macOS, watchOS, and tvOS applications. With its powerful features, intuitive syntax, and seamless integration with Xcode, SwiftUI offers a simpler and more efficient approach to app development. Whether you’re a seasoned developer or just starting your coding journey, SwiftUI empowers you to create stunning, responsive, and user-friendly interfaces with ease.
SwiftUI: The Modern Way to Build UIs
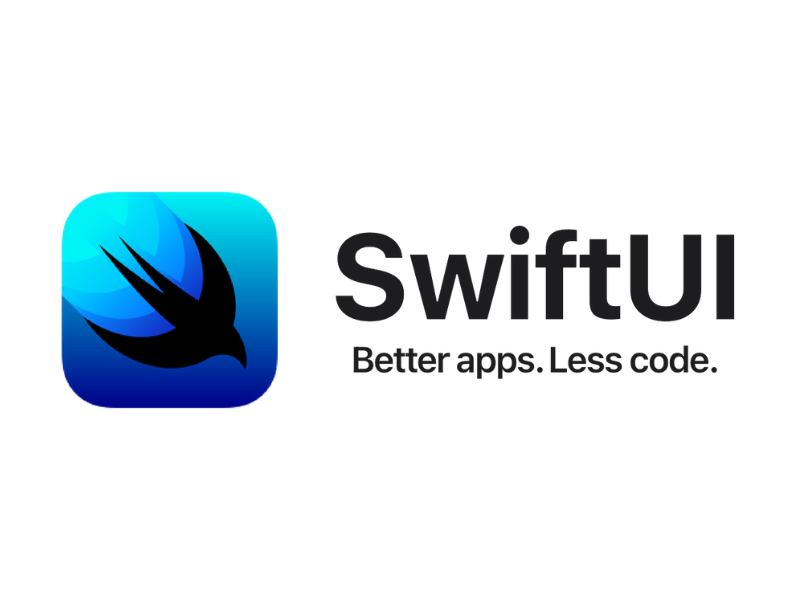
For years, developers relied on UIKit, Apple’s previous UI framework, to design interfaces for their iOS apps. While UIKit was a robust and reliable solution, it often involved writing lengthy code and dealing with complex layout constraints. SwiftUI, on the other hand, embraces a declarative style of programming that allows developers to define what their UI should look like, and the framework automatically handles the rest.
Benefits of SwiftUI
- Simplicity: SwiftUI replaces cumbersome code with intuitive, readable syntax, making it easier to understand and maintain UI layouts.
- Live Preview: With SwiftUI’s live preview feature in Xcode, developers can instantly see the changes they make to their UI code without the need for manual rebuilding or recompiling.
- Cross-Platform Support: SwiftUI provides a unified codebase for creating apps across Apple’s platforms, eliminating the need to write separate UI code for each platform.
- Interactive Design: SwiftUI enables developers to experiment and interact with their UI designs in real-time, with live editing capabilities and interactive components.
- Swift Integration: Being a part of the Swift ecosystem, SwiftUI integrates seamlessly with existing Swift code, allowing developers to leverage the full power of the language.
- Native Performance: SwiftUI leverages Apple’s native frameworks, delivering superior performance and faster rendering compared to traditional approaches.
Getting Started with SwiftUI
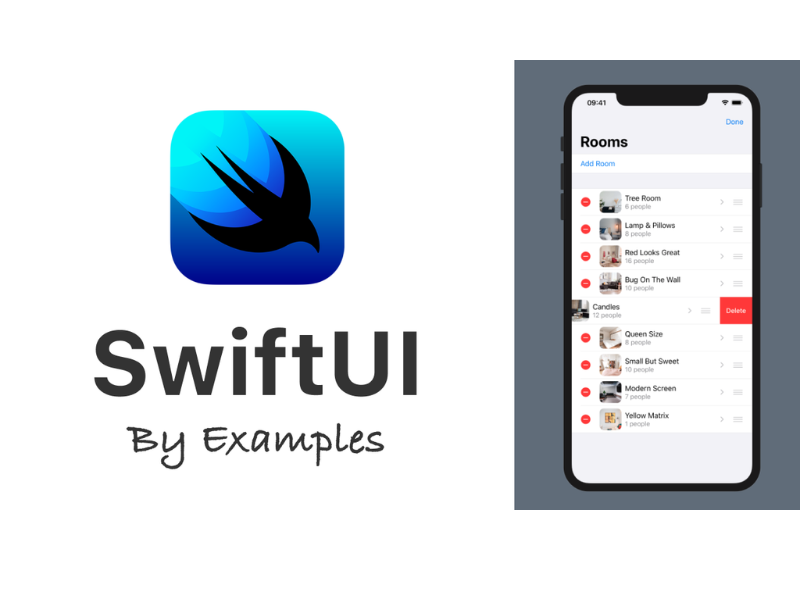
Now that you have an overview of SwiftUI and its advantages, let’s dive into the essentials of getting started with this game-changing framework.
Installing Xcode and SwiftUI
To begin your SwiftUI journey, you’ll need Xcode, Apple’s integrated development environment (IDE) tailored for macOS and iOS app development. Xcode includes all the necessary tools and resources, including SwiftUI, to start building your first SwiftUI-enabled app.
To install Xcode, simply visit the App Store on your Mac, search for Xcode, and click the “Get” button to initiate the download and installation process. Once installed, open Xcode and create a new project. Select the SwiftUI template to generate a basic SwiftUI project structure.
Understanding SwiftUI’s Core Concepts
Views
Views are the building blocks of SwiftUI interfaces. Every visible element on the screen is represented as a view, such as buttons, labels, text fields, and more. Unlike UIKit, where views were based on a hierarchy of UIViews, SwiftUI views are lightweight structs that conform to the View
protocol.
Modifiers
Modifiers are at the heart of SwiftUI’s declarative syntax. Modifiers allow you to change the appearance, behavior, and layout of views. You can chain multiple modifiers together to create complex UI designs effortlessly. For example, you can change the font color of a text view and add a shadow effect with just a few lines of code.
Stacks
Stacks, or layout containers, are essential for organizing and arranging views in SwiftUI. There are two types of stacks: horizontal and vertical. Horizontal stacks arrange views horizontally from leading to trailing, while vertical stacks stack views vertically from top to bottom. Stacks provide automatic alignment, spacing, and resizing of views.
State and Data Flow
State management is crucial in any app, and SwiftUI introduces a new way of handling state through the @State
property wrapper. When the state of a view changes, SwiftUI triggers a re-rendering of that view automatically. This reactive approach ensures that your UI remains synchronized with the underlying data.
Exploring SwiftUI in Action
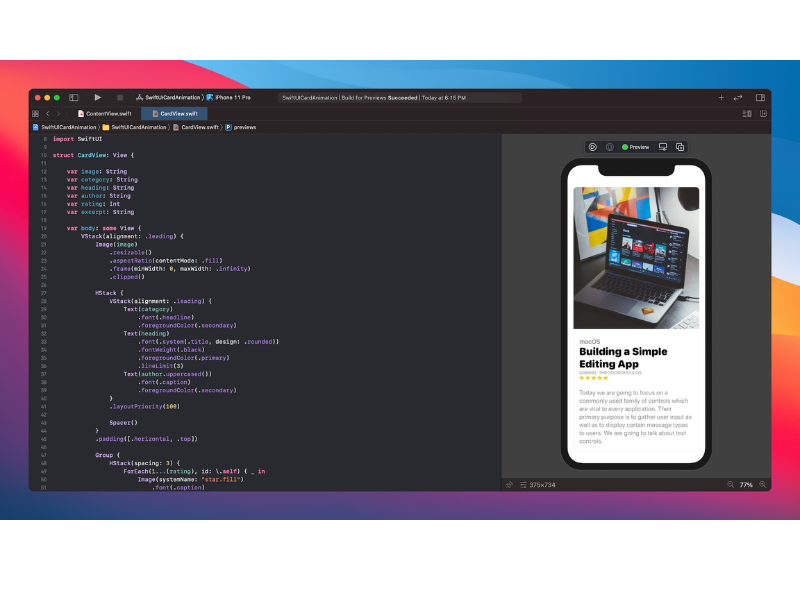
Now that you have a solid understanding of SwiftUI’s core concepts, let’s explore some practical examples to see how the framework simplifies UI development and empowers you to create visually stunning and interactive apps.
Building a To-Do List App
Let’s start by building a simple to-do list app using SwiftUI. First, we’ll create a list view to display the tasks. Each task will be represented by a text view inside a list row.
“`swift struct ContentView: View { var tasks = [“Buy groceries”, “Finish report”, “Walk the dog”]
var body: some View {
List(tasks, id: \.self) { task in
Text(task)
}
}
}
<p>In just a few lines of code, we've created a list view that dynamically displays our tasks. SwiftUI handles all the list rendering and layout automatically, allowing us to focus on the logic and content.</p>
<h4>Customizing the Appearance</h4>
<p>To customize the appearance of our to-do list, we can apply modifiers to the SwiftUI views. Let's add a background color and a rounded corner to the list rows.</p>
<p>```swift
struct ContentView: View {
var tasks = ["Buy groceries", "Finish report", "Walk the dog"]</p>
var body: some View {
List(tasks, id: \.self) { task in
Text(task)
.padding()
.background(Color.blue)
.cornerRadius(10)
}
}
<p>}
With just a few additional lines of code, we’ve transformed our list view into an attractive and visually appealing interface.
Frequently Asked Questions (FAQs)
Can I use SwiftUI with existing UIKit projects?
Yes, SwiftUI is fully compatible with existing UIKit projects. You can start integrating SwiftUI views into your UIKit app gradually, allowing for a smooth transition from UIKit to SwiftUI.
Can I use SwiftUI for macOS development?
Absolutely! SwiftUI is designed to provide a unified framework for developing apps across all Apple platforms, including macOS. With SwiftUI, you can create beautiful and responsive interfaces for your macOS apps effortlessly.
Does SwiftUI support advanced animations and transitions?
Yes, SwiftUI offers a powerful set of tools for creating advanced animations and transitions. With SwiftUI’s built-in animation features, you can easily add eye-catching effects, interactive gestures, and fluid transitions to your app interfaces.
Is SwiftUI suitable for beginner developers?
Yes, SwiftUI is beginner-friendly and a great choice for developers starting their journey in app development. Its intuitive syntax and live preview functionality make it easier for beginners to grasp the concepts and see their UI changes in real-time.
Conclusion
SwiftUI represents the future of app development, offering a simpler, faster, and more enjoyable way to create remarkable user interfaces across Apple’s platforms. With its declarative syntax, extensive modifier library, and seamless integration with Xcode, SwiftUI empowers developers to unleash their creativity and build visually stunning and user-friendly apps. So why wait? Dive into SwiftUI today and experience the freedom and efficiency it brings to your app development journey.